Azoth - a developer blog
Quo Vadis ?
Every journey starts with the most important step - the first one. I knew, going into this, that it was going to be a long haul project, but retirement beckons and I'm going to have to find something to do with all this free time, a concept I'm not overly familiar with …
The Goal(s)
The goal is complex, or at least the path towards the goal is complex. One goal is to create an Objective-C driven user interface framework, that I can compile for either Mac, iOS, Windows and Linux, and get all the benefits of Objective C (memory management, blocks, the Foundation framework). The end-result should be skin-able, so I can use it in different scenarios, but also provide a default "professional"-looking interface as its standard appearance.
But this is not the actual goal. The real goal is to write something similar to the original Baldur's Gate game, again in Objective C, and again for all the above operating systems. The UI and non-gameplay sections of that game will be written in a game-skinned version of the framework, providing me with builtins for scrollbars, collection-views ("inventory"), drag-and-drop etc. That's the real goal. As I said, it's going to be a long haul project [grin]
The vision is something similar to AppKit on the Mac. Something that's familiar, if not exactly the same. It's unlikely to be anywhere near as comprehensive as AppKit, and it'll have a lot more limitations, but it ought to provide a base level of functionality that allows fairly complex user interfaces to be constructed.
Getting there
In order to run-test or "dog-food" the framework, once it's minimally functional, I'll be building a tool application (not for release, so it's ok if it still has rough edges or goes the wrong way a few times before finally being useful) to make sure I shake out the bugs and gotchas that will inevitably creep in. I also intend to write small test-apps along the way to test out the major functionality and act as "how-to" guides being very simple cut-down examples.
I mentioned above that the platforms I'm targeting are the Mac/iOS as well as Linux and Windows. To build cross-platform functionality like that is a huge deal in and of itself, so my plan is to use the newly-released SDL3 to be the rendering underpinnings and provide the graphics abstraction…
Platforms
- Windows: there is a build of Objective C that integrates into Visual Studio, and is supported (and supplied) by Microsoft. There is also a port of the GNUstep Foundation framework (which provides modern ObjC bindings like blocks and libdispatch) for this toolset, so in theory it ought to be plug-and-play. It won't be, things aren't that simple but I have got a simple "hello world" type of application with SDL3 and ObjC both working together.
- Mac / iOS: The Mac is actually where I'm building/testing the majority of the code - well all of it, right now - so that's not going to be an issue. iOS ought to "just work", because the only common dependencies are SDL3 and Foundation, both of which iOS has. I'm expecting there to be implications with the virtual keyboard (for example) amongst other issues like sandboxing. I'll cross that bridge when we get there.
- Linux: It would be nice to support Linux too. Again, the dependencies are SDL3 and Foundation - and Linux is the prime port for GNUStep's Foundation, so in theory it ought to just work. Again, my expectations are that theory and practice will … differ a little.
Nomenclature
Why "Azoth" - well although it sounds like it, there's no association with any demon-worshipping going on here. The background is that the last major framework I wrote was an object-relational database interface, and I called it QuickSilver, which was an old name for the element Mercury. I was going to name this framework 'Mercury', but Apple already has a framework called Mercury installed, so that was out. Looking up synonyms for Mercury revealed Azoth, which is another old name for the element. I guess people really liked to name Mercury things… Anyway, that's the background, and as a result I claim the 'AZ' namespace for all the classes, constants etc. So we have AZApplication (not NSApplication), AZWindow, AZView etc. etc.
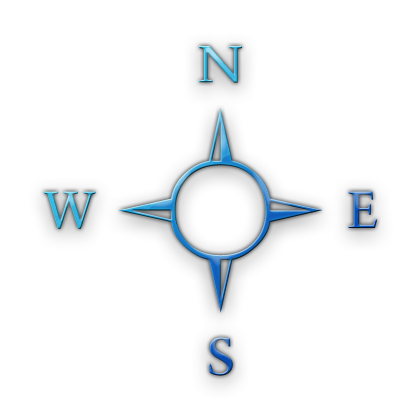